Predefined Array Sorting
Program :-
User -defined Array Sorting
Program :-
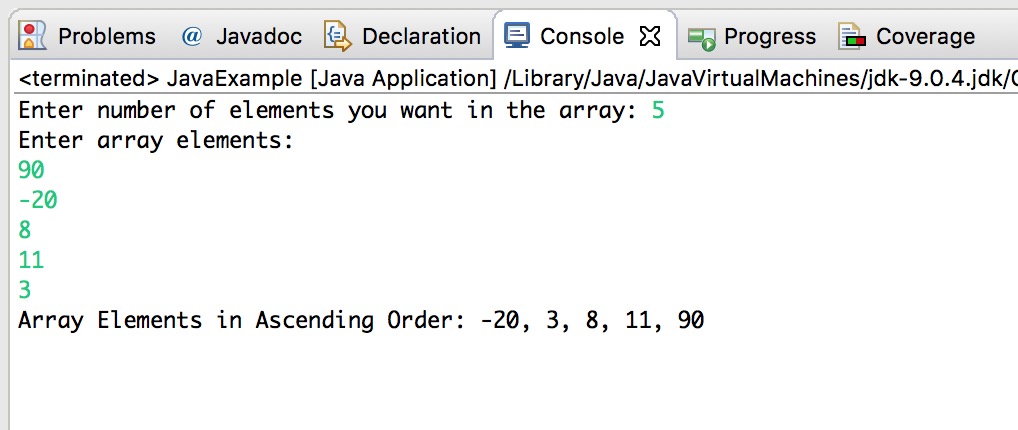
Program :-
import java.util.Arrays; public class SortExample { public static void main(String[] args) { // Our arr contains 8 elements int[] arr = {13, 7, 6, 45, 21, 9, 101, 102}; Arrays.sort(arr); System.out.printf("sorted : %s", Arrays.toString(arr)); } }
User -defined Array Sorting
Program :-
import java.util.Scanner; public class SortFun { public static void main(String[] args) throws Exception { int count, temp; Scanner scan = new Scanner(System.in); System.out.print("Enter number of elements you want in the array: "); count = scan.nextInt(); int num[] = new int[count]; System.out.println("Enter array elements:"); for (int i = 0; i < count; i++) { num[i] = scan.nextInt(); } scan.close(); for (int i = 0; i < count; i++) { for (int j = i + 1; j < count; j++) { if (num[i] > num[j]) { temp = num[i]; num[i] = num[j]; num[j] = temp; } } } System.out.print("Array Elements in Ascending Order: "); for (int i = 0; i < count - 1; i++) { System.out.print(num[i] + ", "); } System.out.print(num[count - 1]); } }
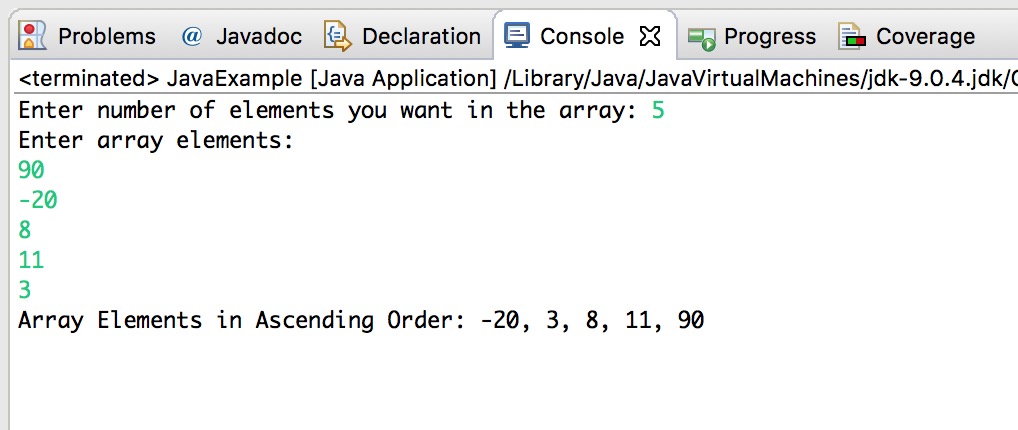