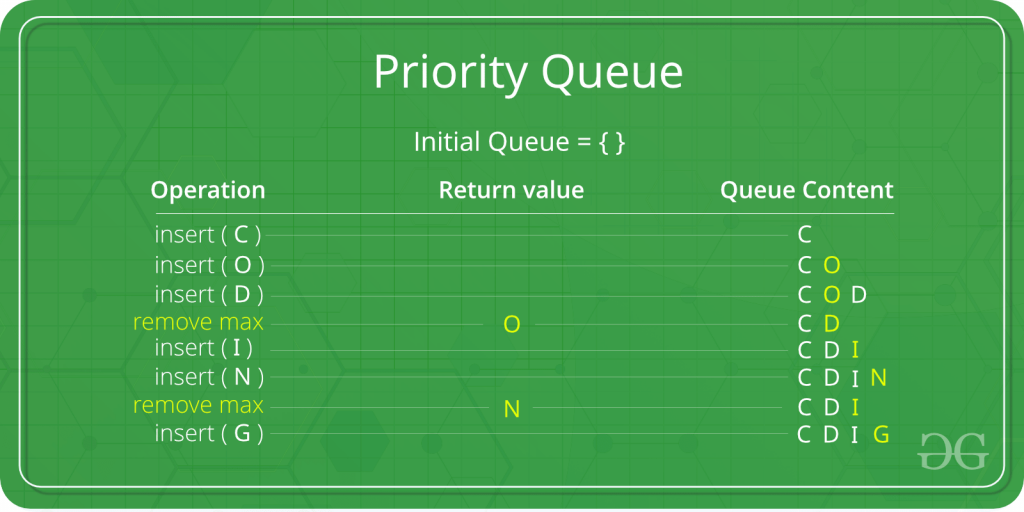
#include<stdio.h>
#include<stdlib.h>
typedef struct node
{
int data;
struct node *next;
}node;
typedef struct
{
node *start;
}LL;
void enqueue(LL *l,int ele)
{
node *newrec,*p;
newrec=(node*)malloc(sizeof(node));
newrec->data=ele;
if(l->start==NULL)
{
l->start=newrec;
newrec->next=NULL;
}
else
{
p=l->start;
while(p->next!=NULL)
{
p=p->next;
}
p->next=newrec;
newrec->next=NULL;
}
}
int dequeue(LL *l)
{
int max;
node *p,*q,*i;
if(l->start==NULL)
{
return -1;
}
else
{
max=l->start->data;
p=l->start;
q=l->start;
i=l->start->next;
while(i!=NULL)
{
if(i->data>max)
{
max=i->data;
p=i;
}
i=i->next;
}
if(l->start->data==max)
{
l->start=q->next;
q->next=NULL;
return q->data;
}
while(q->next!=p)
{
q=q->next;
}
q->next=p->next;
return max;
}
}
void display(LL *l)
{
node *p;
if(l->start==NULL)
{
printf("The linked list is empty\n");
return;
}
else
{
p=l->start;
while(p!=NULL)
{
printf("%d \t",p->data);
p=p->next;
}
printf("\n");
}
}
int main()
{
LL l;
l.start=NULL;
int ch,ele;
while(1)
{
printf("Menu\n1.Enqueue\n2.Dequeue\n3.Display\n4.Exit");
printf("Enter the choice\n");
scanf("%d",&ch);
if(ch==4)
{
break;
}
switch(ch)
{
case 1 : printf("Enter the element to insert\n");
scanf("%d",&ele);
enqueue(&l,ele);
display(&l);
break;
case 2 : if(l.start==NULL)
printf("The linked list is empty\n");
else
{
ele=dequeue(&l);
printf("%d is the deleted element\n",ele);
display(&l);
}
break;
case 3 : display(&l);
break;
default : printf("The choice is invalid\n");
}
}
return 0;
}