In this article I will discuss how to connect MySQL Database on different servers and also give an overview of connecting Database using PDO.
- Connect MySQL using Localhost Server
- Connect MySQL using Cloudways Server
- Connect MySQL using PDO
- Connect MySQL using Remote MySQL
Create MySQL Database at the Localhost
First, let me tell you what PHPMyAdmin is. It is a control panel from where you can manage your database that you have created. Open your browser and go to localhost/PHPMyAdmin or click “Admin” in XAMPP UI.
When you first installed XAMPP, it only created the username for it to be accessed, you now have to add a password to it by yourself. For this, you have to go to User account where the user is same as the one shown in this picture:

Now click Edit privileges and go to Change Admin password, type your password there and save it. Remember this password as it will be use to connect to your Database.
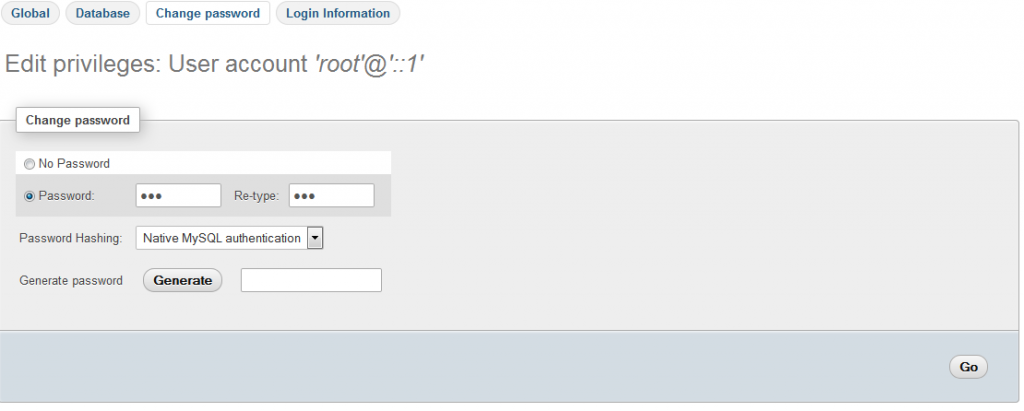
Note: It is not necessary to change password to access databases on local host. It is a good practice and that is why we have used a password.
Create Database
Now return to the homepage of phpmyadmin. Click New button to create a new database.

In the new window, name your database as per your need, I am naming it “practice”. Now select Collation as utf8_general_ci, as we are using it for learning purposes and it will handle all of our queries and data that will be covered in this tutorial series. Now click on Create and your database will be created.
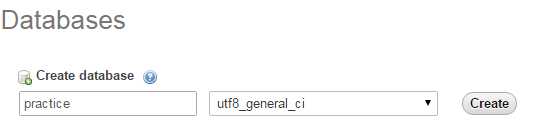
The newly created database will be empty now, as there are no tables in it. I will be covering that in the upcoming series where we will learn how to create tables and insert data in it. In this tutorial, we are going to connect this database to a localhost using PHP.

Create a Folder in htdocs
Now, locate the folder where you installed XAMPP and open htdocs folder (usually c:/xampp). Create a new folder inside c:/xampp/htdocs/ and name it “practice” we will place web files in this folder. Why we have created folder in htdocs? XAMPP uses folders in htdocs to execute and run your PHP sites.
Note: If your using WAMP, then add your practice folder in c:/wamp/www folder.
Create Database Connection File In PHP
Create a new php file and name it db_connnection.php and save it. Why am I creating a separate database connection file? Because if you have created multiple files in which you want to insert data or select data from the databases, you don’t need to write the code for database connection every time. You just have to include it by using PHP custom function include (include ‘connection.php’) on the top of your code and call its function and use it. It also helps when you are moving your project location from one PC to another and you have to change the values on the single file and all the changes will be applied to all the other files automatically. Write the following code in your db_connection file.
- <?php
- function OpenCon()
- {
- $dbhost = "localhost";
- $dbuser = "root";
- $dbpass = "1234";
- $db = "example";
- $conn = new mysqli($dbhost, $dbuser, $dbpass,$db) or die("Connect failed: %s\n". $conn -> error);
- return $conn;
- }
- function CloseCon($conn)
- {
- $conn -> close();
- }
- ?>
Here is the explanation of the variable that we have used in our db_connection file:
- $dbhost will be the host where your server is running it is usually localhost.
- $dbuser will be the username i.e. root and $dbpass will be the password which is the same that you used to access your phpmyadmin.
- $dbname will be the name of your database which we have created in this tutorial.
Create new php file to check your database connection
Create a new php file to connect to your database. Name it index.php and add this code in this file.
- <?php
- include 'db_connection.php';
- $conn = OpenCon();
- echo "Connected Successfully";
- CloseCon($conn);
- ?>
Run it!
Now open your browser and goto localhost/practice/index.php and you should see this screen:

Confirmation Message
Congratulations! You’ve successfully connected your database with your localhost! If you are not able to see this screen, then check if you have done everything right in your db_connection.php file.
Create MySQL Database at Cloudways Server
For the purpose of this tutorial, I assume that you have a PHP application installed on a web server. My setup is:
- PHP 7.2
- MySQL
Since I wish to focus on the topic under discussion, I decided to host my PHP application on Cloudways managed servers because I get a highly optimized hosting stack and no server management hassles. You can try out Cloudways for free by signing for an account and then following this simple GIF for setting up the server and the PHP application.
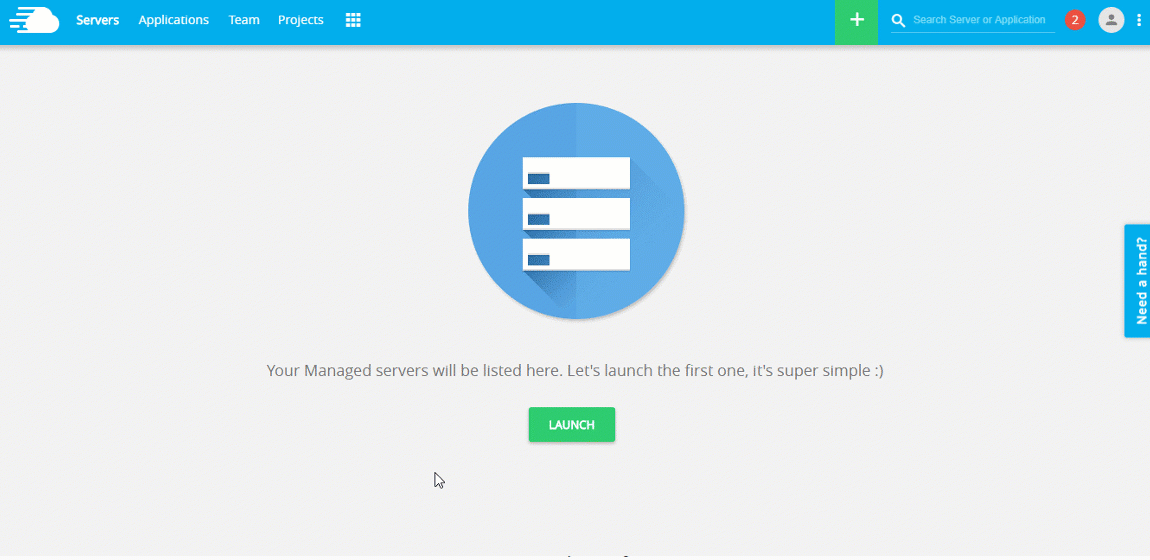
After successfully launch PHP Application on Cloudways go in the application tab and check the details of database and also you click on button to launch database manager.
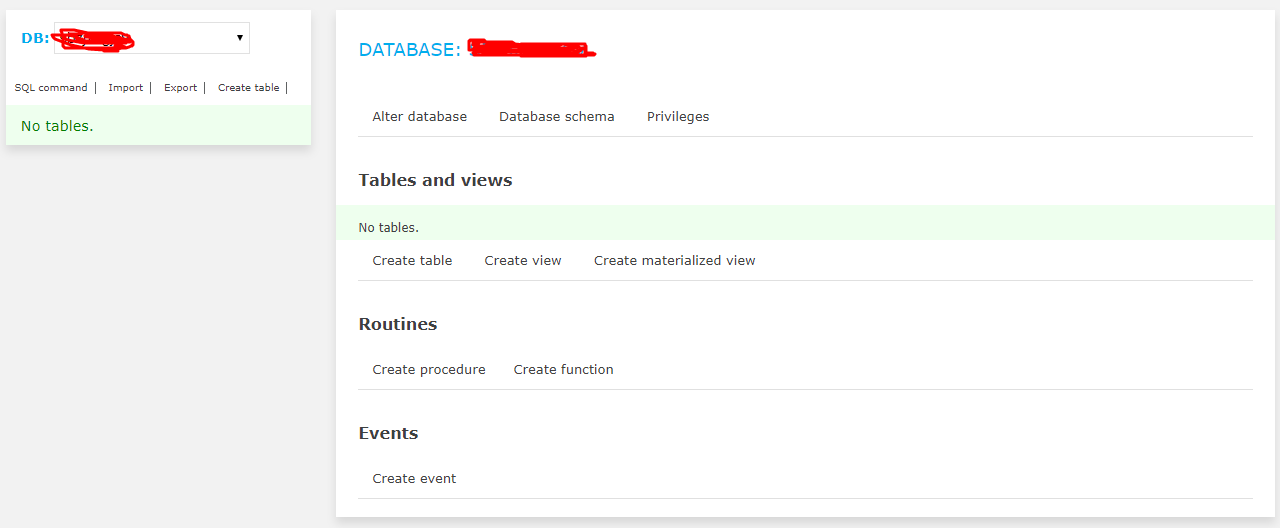
Create Database Connection
The first order of the business is to set up a connection to the database. For this use the function mysql_connect. This function returns a pointer (also known as a database handle) to the database connection. This handle will be used in the code later on. Once you have the handle, remember to add your database credentials.
Create a new php file and name it db_connnection.php and save it. Why am I creating a separate database connection file? Because if you have created multiple files in which you want to insert data or select data from the databases, you don’t need to write the code for database connection every time. You just have to include it by using PHP custom function include (include ‘connection.php’) on the top of your code and call its function and use it.
At this point you have the option of either using MySQLi procedural connection query or PHP PDO based database connection:
MySQLi Procedural Query
- <?php
- $servername = "localhost";
- $username = "username";
- $password = "password";
- $db = "dbname";
- // Create connection
- $conn = mysqli_connect($servername, $username, $password,$db);
- // Check connection
- if (!$conn) {
- die("Connection failed: " . mysqli_connect_error());
- }
- echo "Connected successfully";
- ?>
Connect MySQL Database with PHP Using PDO
- <?php
- $servername = "localhost";
- $username = "username";
- $password = "password";
- $db = "dbname";
- try {
- $conn = new PDO("mysql:host=$servername;dbname=myDB", $username, $password, $db);
- // set the PDO error mode to exception
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- echo "Connected successfully";
- }
- catch(PDOException $e)
- {
- echo "Connection failed: " . $e->getMessage();
- }
- ?>
Check Connection
- <?php
- include 'db_connection.php';
- echo "Connected Successfully";
- mysqli_close($conn);
- ?>
For PDO Close the Connection like this
- $conn = null;

Remote MySQL
For Remote MySQL connection Log into the Cloudways Platform with your credentials. Click on “Servers” in the top menu bar. Then, click on your target server from the list.
- Next, go to Security menu option in the left menu, and then click the MySQL tab.
- Add the IP address to the “Add IP to Whitelist” text area and click the “Add” button.
- If you have multiple IP addresses, repeat the process.
- Once you are done, click the “Save Changes” button to finalize the changes
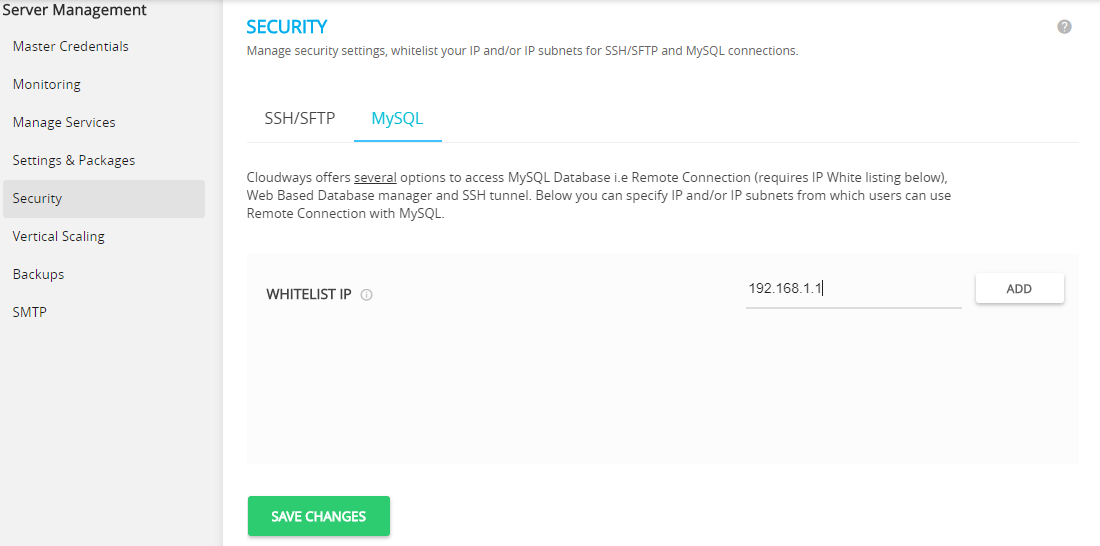
After successfully , set the IP Whitelisting set the IP address in connection and run the query.
Conclusion
MySQL is the backbone of any web project. Whether its a CMS or an online task tracking platform, you need a MySQL database(s) to keep track of app and user information and server data to the application processes.
In this tutorial, we have learned two things:
- How to create a new database
- How to connect MySQL database with PHP
We now have our databases ready for data-entry by creating tables in the newly created databases.
If you need help in using MySQL in your projects, do leave a comment below.
In the next installment of this MySQL series, I will introduce MySQL data types. Here is a short introduction and list of major topics in this MySQL series.
Turbo-charged MySQL Hosting
Cloudways provides a Managed Web Hosting Stack based on MySQL, PHP, Varnish HTTP Accelerator, Nginx and Apache web servers, and Memcached and Redis cache. Your website load time can improve by up to 100% on the Cloudways Platform.